介绍
注意:此模块仅作推送使用,不可交互 !!!
统一化推送服务Nodejs API. 已支持钉钉, Discord, 邮件, 飞书, PushDeer, PushPlus, QQ, QQ 频道机器人, Server 酱, Showdoc Push, Telegram Bot, 企业微信群机器人, 息知, WxPusher, NowPush, iGot, Chanify, Bark, Push, Slack, Pushback, Zulip, RocketChat, Gitter等平台.
已支持平台
- QQ(go-cqhttp)– GoCqhttp
QQ(Qmsg)– Qmsg- QQ 频道机器人– QqChannel
- 钉钉群机器人– DingTalk
- Discord– Discord
- 邮件– Mail
- 飞书群机器人– FeiShu
- 企业微信– WorkWeixin
- 企业微信群机器人– WorkWeixinBot
- Telegram Bot– TelegramBot
- PushDeer– PushDeer
- PushPlus– PushPlus
- Server 酱– ServerChanTurbo
- Showdoc Push– Showdoc
- 息知– Xizhi
- WxPusher– WxPusher
NowPush– NowPush- iGot– IGot
- Chanify– Chanify
- Bark– Bark
- GoogleChat– GoogleChat
- Push– Push
- Slack– Slack
- Pushback– Pushback
- Zulip– Zulip
- RocketChat– RocketChat
- Gitter– Gitter
- Pushover– Pushover
安装
npm install all-pusher-api -S
使用
Example
多平台推送
const { PushApi } = require('all-pusher-api'); // 多平台同时推送
(async () => {
console.log((await new PushApi([
{
name: 'ServerChanTurbo',
config: {
key: {
token: '******'
}
}
},
{
name: 'PushDeer',
config: {
key: {
token: '******'
}
}
},
{
name: 'WxPusher',
config: {
key: {
token: '******',
uids: ['******']
}
}
}
])
.send({ message: '测试文本' })).map((e) => (e.result.status >= 200 && e.result.status < 300) ? `${e.name} 测试成功` : e));
})();
单平台推送
(async () => {
// Example 1
const { PushApi } = require('all-pusher-api'); // 多平台同时推送
const result = await new PushApi([
{
name: 'ServerChanTurbo',
config: {
key: {
token: '******'
}
}
}
])
.send({ message: '测试文本' });
console.log(result.map((e) => (e.result.status >= 200 && e.result.status < 300) ? `${e.name} 测试成功` : e));
// Example 2
const { WxPusher } = require('all-pusher-api/dist/WxPusher'); // 单平台推送可选
const wxPusherResult = await new WxPusher({
token: '******',
uids: ['******']
})
.send({ message: '测试文本' });
console.log(wxPusherResult);
})();
extraOptions
(async () => {
const { DingTalk } = require('all-pusher-api/dist/DingTalk');
const result = await new DingTalk({
key: {
token: '******',
secret: '******'
}
})
.send({
message: '这条消息@了所有人',
extraOptions: {
isAtAll: true
}
});
console.log(result);
})();
customOptions
这里以钉钉为例
(async () => {
const { DingTalk } = require('all-pusher-api/dist/DingTalk');
const result = await new DingTalk({
key: {
token: '******',
secret: '******'
}
})
.send({
customOptions: {
"msgtype": "actionCard",
"actionCard": {
"title": "我 20 年前想打造一间苹果咖啡厅, 而它正是 Apple Store 的前身",
"text": "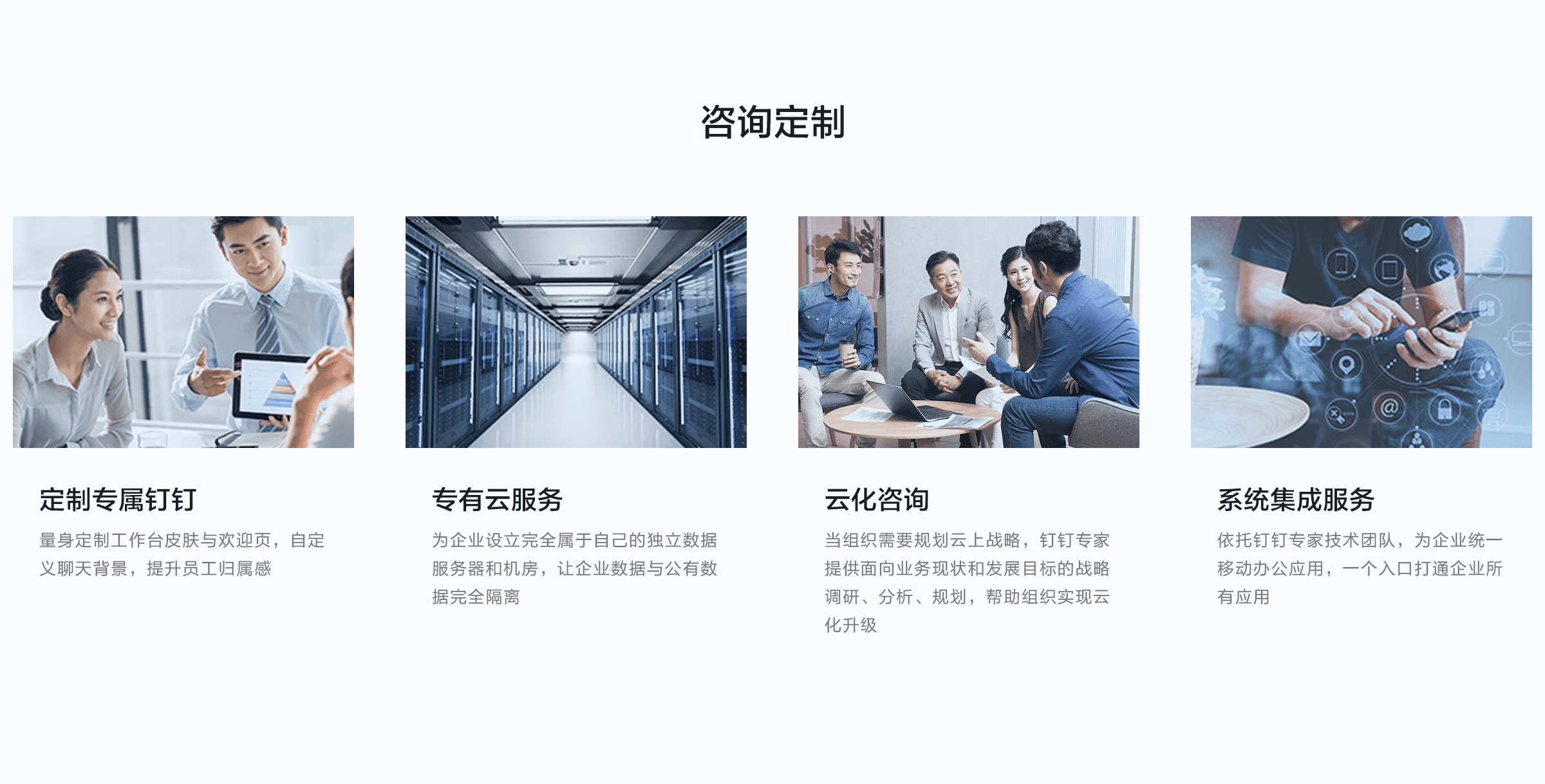 \n\n #### 乔布斯 20 年前想打造的苹果咖啡厅 \n\n Apple Store 的设计正从原来满满的科技感走向生活化, 而其生活化的走向其实可以追溯到 20 年前苹果一个建立咖啡馆的计划",
"btnOrientation": "0",
"btns": [
{
"title": "内容不错",
"actionURL": "https://www.dingtalk.com/"
},
{
"title": "不感兴趣",
"actionURL": "https://www.dingtalk.com/"
}
]
}
}
});
console.log(result);
})();
自定义接口
(async () => {
const { Custom } = require('all-pusher-api/dist/Custom'); // 自定义接口只能通过此方法引入
const { createHmac } = require('crypto');
const sign = () => {
const timestamp = new Date().getTime();
const secret = '******';
const stringToSign = `${timestamp}\n${secret}`;
const hash = createHmac('sha256', secret)
.update(stringToSign, 'utf8')
.digest();
return `timestamp=${timestamp}&sign=${encodeURIComponent(hash.toString('base64'))}`;
};
console.log(await new Custom({
url: `https://oapi.dingtalk.com/robot/send?access_token=******&${sign()}`,
success: {
key: 'responseData.errcode',
value: 0
}
}).send({
msgtype: 'text',
text: {
content: '测试文本'
}
}));
/* 返回值
{
status: 200,
statusText: 'Success',
extraMessage: <AxiosResponse>
}
*/
});
参数
pusherConfig
这部分写的比较乱,建议配合参数生成器使用!
const pusher = new WxPusher(pusherConfig);
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
token | string | null | 大部分平台的授权token, 如果有授权信息有多个, 请使用key |
baseUrl | string | null | 对于部分支持搭建服务端的平台, 如果使用自建服务端, 需配置此选项 |
webhook | string | null | Discord ,企业微信机器人 ,RocketChat 和GoogleChat 的 webhook 地址, 该平台请使用webhook 而不是token |
userId | string | null | Pushback 平台的 User_id |
chat_id | string | null | Telegram 平台的 chat_id |
email | string | null | Zulip 平台的 bot email |
domain | string | null | Zulip 平台的 domain |
to | Array<number\|string> | null | Zulip 平台的发送对象 |
roomId | string | null | Gitter 平台发送对象的 roomid |
baseUrl | string | null | go-cqhttp 的http通信地址, 以http:// 或https:// 开头 |
user_id | number | null | 使用 go-cqhttp 推送时的目标 QQ 号, 此参数与group_id ,channel_id 二选一 |
group_id | number | null | 使用 go-cqhttp 推送时的目标群号, 此参数与user_id ,channel_id 二选一 |
channel_id | string | null | 使用 go-cqhttp 推送时的目标频道ID, 此参数与user_id ,group_id 二选一, 且必须与guild_id 同时存在 |
guild_id | string | null | 使用 go-cqhttp 推送时的目标子频道ID, 此参数必须与channel_id 同时存在 |
corpid | string | null | 企业微信群机器人的corpid |
agentid | string | null | 企业微信群机器人的agentid |
secret | string | null | 钉钉、飞书加签的密钥[可选]/企业微信群机器人的secret |
touser | string | null | 企业微信群机器人指定接收消息的成员, 也可在sendOptions中配置 |
uids | Array<string> | null | WxPusher 发送目标的 UID, 也可在sendOptions中配置 |
topicIds | Array<number> | null | WxPusher 发送目标的 topicId, 也可在sendOptions中配置 |
appID | string | null | QQ频道机器人的 ID, 使用QQ频道推送时此选项为必选 |
token | string | null | QQ频道机器人的 token, 使用QQ频道推送时此选项为必选 |
sandbox | boolean | false | 使用QQ频道推送时是否启用沙箱, 可选 |
channelID | string | null | QQ频道的子频道 ID, 使用QQ频道推送时此选项为必选 |
user | string | null | Pushover 的 user key, 使用 Pushover 推送时此选项为必选 |
key | object | null | 以上参数都可以放到key 中,示例 |
-key.host | string | null | 邮件发送服务器地址, 使用邮件推送时此选项为必选 |
-key.port | number | null | 邮件发送服务器端口, 使用邮件推送时此选项为必选 |
-key.secure | boolean | false | 邮件发送服务器是否启用TLS/SSL, 可选 |
-key.auth | object | null | 邮件发送服务器的验证信息, 使用邮件推送时此选项为必选 |
-key.auth.user | string | null | 邮件发送服务器的用户名, 使用邮件推送时此选项为必选 |
-key.auth.pass | string | null | 邮件发送服务器的密码, 使用邮件推送时此选项为必选 |
proxy | object | null | 代理配置, 可选, 部分支持 |
-proxy.enable | boolean | false | 是否启用代理 |
-proxy.protocol | string | 'http' | 代理协议 |
-proxy.host | string | null | 代理主机地址 |
-proxy.port | number | null | 代理端口 |
-proxy.username | string | null | 代理用户名 |
-proxy.password | string | null | 代理密码 |
CustomConfig
自定义接口
const customPusher = new Custom(CustomConfig);
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
url | string | null | 请求链接, 必需 |
method | string | 'POST' | 请求方式, 可选 |
contentType | string | 'application/json' | 发送的数据类型, 等同于hreders['Content-type'] |
headers | AxiosRequestHeaders | null | 请求头, 可选 |
success | object | null | 推送成功的判断方式, 必需 |
-success.key | string | null | 请看示例 |
-success.value | any | null | 请看示例 |
key | object | null | 以上参数都可以放到key中 |
proxy | object | null | 代理配置, 同上 |
pushersConfig
const pushers = new PushApi(pushersConfig);
const pushersConfig: Array<{
name: string,
config: pusherConfig
}>
sendOptions
const result = await pusher.send(sendOptions);
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
message | string | null | 推送的消息内容,message 与customOptions 至少要有一个 |
title | string | null | 部分平台支持消息标题, 不填则自动提取message 第一行的前10个字符 |
type | string | 'text' | 仅支持text ,markdown ,html . 具体平台支持情况请查看支持的消息类型 |
extraOptions | object | null | 附加内容, 此对象中的内容会附加到请求体中,示例 |
customOptions | object | null | 自定义请求内容, 推送时会POSTcustomOptions ,示例 |
customSendOptions
const result = await customPusher.send(customSendOptions);
customSendOptions
会直接作为请求体发送, 具体请查看示例.
pushersSendConfig
const results = await pushers.send(pushersSendConfig);
const pushersSendConfig: Array<{
name: string
config: pushersSendConfig
}>
返回值
result
constresult= await pusher.send(sendOptions);
参数 | 类型 | 默认值 | 说明 |
---|---|---|---|
status | number | null | 状态码 |
statusText | string | null | 状态说明文本 |
extraMessage | AxiosResponse | Error | null |
results
constresults= await pushers.send(pushersSendConfig);
const results: Array<{
name: string
result: result
}>
支持的消息类型
所有平台支持均纯文本(
text
)格式消息, 大部分支持markdown
格式消息, 部分支持html
格式消息
markdown*
为支持html
格式不支持markdown
格式消息时自动将markdown
转换为html
格式
other
为部分平台支持特殊格式的消息, 可通过customOptions
传入参数, 具体参数请查看相应平台的文档
- Showdoc:
text
- Pushover:
text
- QQ(go-cqhttp):
text
,other
- Qmsg:
text
,other
- Discord:
text
,other
- 飞书:
text
,other
- NowPush:
text
,other
- Chanify:
text
,other
- Bark:
text
,other
- Server酱Turbo:
text
,markdown
- 息知:
text
,markdown
- PushDeer:
text
,markdown
,other
- QQ频道:
text
,markdown
,other
- 企业微信:
text
,markdown
,other
- 企业微信群机器人:
text
,markdown
,other
- 钉钉:
text
,markdown
,other
- TelegramBot:
text
,markdown
,html
- 邮件:
text
,markdown*
,html
- PushPlus:
text
,markdown*
,html
- WxPusher:
text
,markdown*
,html
状态码
0
-Missing Parameter: ***
: 缺少必要参数10
-Missing Options
: 缺少发送消息配置11
-Unknown Error
: 未知错误200
-Success
: 推送成功201
-Waiting
: 待审核100
-Error
: 请求发送成功, 服务器返回错误信息101
-No Response Data
: 请求发送成功, 但没有接收到服务器返回的数据102
-Request Error
: 请求发送失败, 一般是网络问题103
-Options Format Error
: 参数格式错误104
-Get "***" Failed
: 获取参数失败140
-Check Sign Failed
: 签名校检失败